Schrödinger's Website
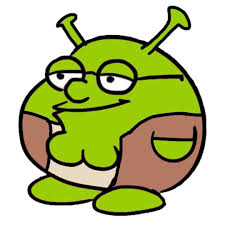

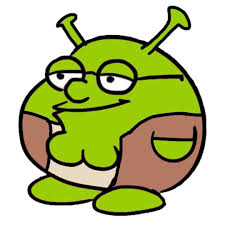
The Double-Slit Experiment
Ever heard of the double slit experiment? It's an experiment that shows that particles can behave like a wave:
But the crazy thing is, when you do this:
They do this:
The simple act of observing something changes how it behaves.
Without getting to into the nitty-gritty, the basic idea is that particles behaving like a wave is "quantum behavior". The particle doesn't actually exist in a specific spot, and instead, is a wave of probabilities. A good way to visualize this is with something that makes actual waves, like water:
But physical objects acting like a wave doesn't make sense?! Well, the universe agrees with you, which is why when you try to watch this "quantum behavior", the particles say "lets act normal" and only show up on two spots on the back of the board.
You can only see the wave-like pattern if you look at the results after the experiment. If you try to watch it happen in real-time, you force the particles to behave normally.
Conclusion: The act of observing something changes how it behaves
The Website
There is a whole rabbit hole you can dive into if you start researching this experiment and the related physics, but thats not what this post is about.
This post is about schrodingers.website, a website which changes behavior when its observed.
Me being frustrated trying to access the website
So what does this mean? Well I designed a beautiful homepage with the most amazing content in the world, which nobody will ever see. Whenever you try to access the website, it causes it to change, until you stop trying to look at it.
I implemented this using two methods:
1. Conditional Rendering
2. Conditional Shutdown
I will show you how I implemented both, and the differences between the two.
Setup
The initial setup for both methods is the same. I used Node.js and Express.js. Make sure to download Node.js if you havent already. I will show you how to implement it locally, which you can then modify if you want to put it on the world wide interwebs.
1. Create your local environment
In your command line, make a directory for your project and go into that directory
mkdir schrodingers-website && cd schrodingers-website
Initialize a new Node.js project
npm init -y
Install required dependencies for your project. Replace your dependencies field in your package.json file with this:
"dependencies": {
"@babel/core": "^7.22.10",
"@babel/preset-env": "^7.22.10",
"@babel/preset-react": "^7.22.5",
"@babel/register": "^7.22.5",
"babel-preset-env": "^1.7.0",
"babel-preset-react": "^6.24.1",
"babel-register": "^6.26.0",
"express": "^4.18.2",
"react": "^18.2.0",
"react-dom": "^18.2.0"
}
The basic dependencies here are react, express, and babel. Babel is going to be used so we can do server side rendering.
Also make sure you have a main field that looks like this:
"main": "index.js"
This allows our server to know that index.js is our home page, which we will soon make impossible to observe.
Once you have the dependencies setup, install them in your project directory:
npm install
2. Set up relevant files
All my files are in the root directory of my project. You can make a src folder and public folder if you want, to start organizing things a little more, but it's not necessary for this project.
index.js: This is YOUR homepage, make it look however you want! It almost feels pointless as nobody will ever see it, but I spent about 1 year making mine.
This is my basic setup, but im keeping my internals a secret:
import React, { Component } from "react";
class HomePage extends Component {
render() {
return (
//Secret Sauce
);
}
}
export default HomePage;
renderer.js: This file helps your server perform server-side rendering, and converts your index.js file into something it can understand
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import HomePage from './index.js';
export function renderHomePage() {
return ReactDOMServer.renderToString(<HomePage />);
}
.babelrc: This file helps configure some stuff related to babel and server-side rendering
{
"presets": [
"@babel/preset-env",
"@babel/preset-react"
]
}
server.js: This file will differ between the conditional rendering implementation and the conditional shutdown implementation, but there are some common elements we can put at the top of this file:
require('@babel/register');
const express = require('express');
const http = require('http');
const path = require('path');
const { renderHomePage } = require('./renderer');
const PORT = 3000;
const app = express();
const server = http.createServer(app);
// Toggle this variable to control the behavior
let schrodingerLogic = true;
Here, I just specify my imports and initialize some variables. I have a boolean called schrodingerLogic, which I can toggle off to observe my homepage while developing. When this variable is on, it will trigger the logic which handles either conditional renderings or conditional shutdowns.
Conditional Rendering
The basic idea behind this method is that I have a conditional system where when a user visits the website, it changes its default rendering (My lifechanging homepage you will never get to see) to be a error page hosted by the server.
Custom Error Page
I like this method because this allows me to have a custom error page, instead of the default one provided by the browser.
Your custom error page can be whatever you want. What I did is take the default error page my web browser provides, put it in a file called errorPage.html and modify it a little:
Original
Mine
If you want to use or modify the error page provided by the browser, just navigate to a website that does not exist, and use inspect element to extract the HTML
Server Logic
Now we will build the body of server.js to handle the conditional rendering. The way I did this is I have two new variables I added to my file:
let isLockdown = false;
let lockdownTimer;
I use these variables to essentially keep track of when my webpage has been observed. The timer is used to tell the server how long it should remain labeled as observed. I have my timer set to one minute.
This takes a lot less work to set up than keeping track of whether its being observed in real-time, as that would require a lot of server-client synchronization, making it very difficult. You would use a package like sockets.io if you wanted to go down this road.
We also want to add a new import statement to the file:
const fs = require('fs');
This import will be used so we can fetch the our custom error page and render it instead.
Now all thats left is to implement app.get
app.get('/', (req, res) => {
});
This function basically tells the website what to do when a user visits it. Since we want to toggle the lockdown functionality on and off, lets start by dividing it into two conditional statements. One where schrodingerLogic is on, and one where schrodingerLogic is off:
app.get('/', (req, res) => {
if (schrodingerLogic) {
}
else {
}
});
We know that if the functionality is off, all we want to do is view the secret homepage, so we use our renderHomePage function to fetch content from index.js and then input it into a HTML format:
app.get('/', (req, res) => {
if (schrodingerLogic) {
}
else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
When the functionality is on, we want to immediately set isLockdown to true, display the error page, and then specify our lockdown duration of 1 minute. We also tie a condition to unlock the website when the timer hits zero:
app.get('/', (req, res) => {
if (schrodingerLogic) {
// Initiate lockdown immediately on request
isLockdown = true;
console.log("Lockdown initiated.");
// Serve the error page to everyone including the user who triggered the lockdown
fs.readFile(path.join(__dirname, 'errorPage.html'), 'utf-8', (err, content) => {
if (err) {
res.status(500).send('Something went wrong!');
return;
}
res.send(content);
});
// Reset after a certain duration
const lockdownDuration = 60000; // E.g., 1 minute; adjust as needed
clearTimeout(lockdownTimer); // Clear any previous timer to avoid overlaps
lockdownTimer = setTimeout(() => {
isLockdown = false;
console.log("Lockdown expired.");
}, lockdownDuration);
}
else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
And thats it! All thats left is to make sure we are listening on the port:
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Our complete file should look something like this:
server.js
require('@babel/register');
const express = require('express');
const http = require('http');
const path = require('path');
const { renderHomePage } = require('./renderer');
const fs = require('fs');
const PORT = 3000;
const app = express();
const server = http.createServer(app);
// Toggle this variable to control the behavior
let schrodingerLogic = true;
let isLockdown = false;
let lockdownTimer;
app.get('/', (req, res) => {
if (schrodingerLogic) {
// Initiate lockdown immediately on request
isLockdown = true;
console.log("Lockdown initiated.");
// Serve the error page to everyone including the user who triggered the lockdown
fs.readFile(path.join(__dirname, 'errorPage.html'), 'utf-8', (err, content) => {
if (err) {
res.status(500).send('Something went wrong!');
return;
}
res.send(content);
});
// Reset after a certain duration
const lockdownDuration = 60000; // E.g., 1 minute; adjust as needed
clearTimeout(lockdownTimer); // Clear any previous timer to avoid overlaps
lockdownTimer = setTimeout(() => {
isLockdown = false;
console.log("Lockdown expired.");
}, lockdownDuration);
} else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
And voila! You have your very own quantum website (using conditional rendering).
Conditional Shutdown
Whats funnier than showing a custom error page when somebody tries to view your website? Yeah thats right, shutting it down completely.
There were some interesting challenges to implementing this version of the website, because when the server is offline, it can't really see who is trying to access it. For this reason, we use the same idea that we did in the conditional rendering version. We shut down the server, and restart it after 1 minute.
Server Logic
We only need to modify server.js to make this version work. Firstly, we want to keep track of our active connections, and write a function to delete them when a user accesses the website:
const connections = {};
server.on('connection', (conn) => {
const key = `${conn.remoteAddress}:${conn.remotePort}`;
connections[key] = conn;
conn.on('close', () => {
delete connections[key];
});
});
The reason we need to do this is because if we just use server.close, the function waits for all users to disconnect before fully shutting down. This means that if you try and access the website, you'll often be caught in a infinite loading loop, as the server is only partially shutdown.
This will allow for proper shutdowns regardless of what users are doing, and quickly direct them to the browser default error page:
Now, similarly to the conditional rendering implementation, we want to make our app.get function. We can set it up with the same if-else statement setup:
app.get('/', (req, res) => {
if (schrodingerLogic) {
}
else {
}
});
Since we want it to show the homepage when the schrodinger logic is off, we can copy and paste the code from the previous implementation, and it will work just fine:
app.get('/', (req, res) => {
if (schrodingerLogic) {
}
else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
When the logic is on, we want to shut down the server for 1 minute when the website is visited. We first delete all the connections, run server.close and tie it to a timeout of 1 minute:
app.get('/', (req, res) => {
if (schrodingerLogic) {
console.log('Someone tried to observe the server!');
// Destroy all active connections
for (const key in connections) {
connections[key].destroy();
}
// Close the server and set it to restart after 1 minute
server.close(() => {
console.log('Server is now offline.');
setTimeout(() => {
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
}, 60000); // 1 minute
});
}
else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
Now the whole file should look like this:
server.js
require('@babel/register');
const express = require('express');
const http = require('http');
const path = require('path');
const { renderHomePage } = require('./renderer');
const PORT = 3000;
const app = express();
const server = http.createServer(app);
// Toggle this variable to control the behavior
let schrodingerLogic = true;
const connections = {};
server.on('connection', (conn) => {
const key = `${conn.remoteAddress}:${conn.remotePort}`;
connections[key] = conn;
conn.on('close', () => {
delete connections[key];
});
});
app.get('/', (req, res) => {
if (schrodingerLogic) {
console.log('Someone tried to observe the server!');
// Destroy all active connections
for (const key in connections) {
connections[key].destroy();
}
// Close the server and set it to restart after 1 minute
server.close(() => {
console.log('Server is now offline.');
setTimeout(() => {
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
}, 60000); // 1 minute
});
}
else {
// If schrodingerLogic is false, always serve the home page
const content = renderHomePage();
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Homepage Title</title>
</head>
<body>
<div id="app">${content}</div>
</body>
</html>
`;
res.send(html);
}
});
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
And there you go! You can now shutdown your website when anybody tries to take a peek. Just like quAaNTuM mEChAnICS!
Thoughts
We are proud to announce that this is probably our most useless product to date, as from a user perspective, they can't even tell the website exists in the first place. But deep down as developers... We know its there. We know all the hard work and love we poured into making a quantum website, and thats all that matters right?
Wrong, thats why I made this blog post so people will know it actually exists.
...Or does it?
Code for this project can be found here!
Do you like unhinged content? Follow me on X! Or Buy me a coffee!